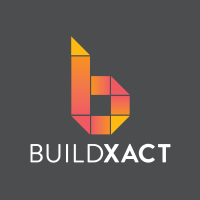
Authentication
Buildxact offers two types of authentication flows:
First-party authentication. If you are a Buildxact customer, accessing your data directly.
Third-party authentication. If you are offering a SaaS platform of your own and you want Buildxact users to be able to connect their accounts to your platform.
You do not need to send the Subscription Key to any of these requests.
1. First Party authentication
This section describes first-party authentication (a Buildxact customer accessing their own data) only. If you are building a third-party OAuth flow, please see the Third-Party Authentication section instead.
Before you can implement this authentication, you will need to contact Buildxact Support to provide a client_id and client_secret for you to access the authentication endpoint.
Buildxact provides an OAuth-based token mechanism, with long-lived refresh tokens and short-lived bearer tokens (also known as “access tokens”).
When you obtain an access token, it is valid for a period of time and can be used until it expires. Please code your logic to check the expires_in field shown below, and persist the token until it expires (as per OAuth standards, the expires_in field is the number of seconds that the token is valid for).
When the access token expires (or before), you can get a replacement token by calling the Refresh Token endpoint to replace it.
Buildxact will not allow you to have more than 200 active refresh tokens; if you generate more than this, the oldest one will automatically be deactivated.
Do not attempt to request a new token for every API request – please use the one you already have if it is still valid!
AUTHENTICATION REQUEST
POST https://api.buildxact.com/oauth/token
Content-Type: application/x-www-form-urlencoded
Body: username=
<username>
&password=
<password>
&grant_type=password&client_id=
<clientid>
&client_secret=
<clientsecret>
Body Parameters
<username> Your Buildxact user name
<password> Your Buildxact password
<clientid> API client id as provided by Buildxact
<clientsecret> API client secret as provided by Buildxact
RESPONSE
{
"access_token": "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJi...",
"token_type": "bearer",
"expires_in": 86399,
"refresh_token": "42f3df53a67c42d9b2246f95f086eecc"
}
expires_in: Total seconds access token is valid for
refresh_token: Can be used later to obtain a new access token without logging in again. We advise you to securely store this refresh token to avoid having to re-authenticate with username & password.
Calling the APIs with the Access Token
For all calls to the Buildxact APIs, you must supply the access token value as an Authorization Header on the request:
Authorization: Bearer <access_token>
Ensure the value for the Authorization header begins with ‘bearer’, followed by a space, then the access_token value you received.
Obtaining an impersonation token (franchises only)
You may have access to more than one tenant's data in Buildxact. This is likely to be the case if you run a franchise business or a larger business consisting of multiple sub-tenants. When requesting a token, you can specify the ID of a tenant you would like access to other than the one your user account is related to. To facilitate this, pass in the tenant ID you are requesting access to along with your login credentials.
AUTHENTICATION REQUEST
POST https://api.buildxact.com/oauth/token
Content-Type: application/x-www-form-urlencoded
Body: username=
<username>
&password=
<password>
&grant_type=password&client_id=
<clientid>
&client_secret=
<clientsecret>
&tenant_id=
<tenantId>
Body Parameters
<username> Your Buildxact user name
<password> Your Buildxact password
<clientid> API client id as provided by Buildxact
<clientsecret> API client secret as provided by Buildxact
<tenantId> Tenant Id Guid you are requesting access to.
If you are unsure of the Tenant Id's that you can access, take these steps first:
1. Obtain a login token as per normal (without tenant Id)
2. Using that token, make a GET request to /accounts/tenants which will return the tenants you have access to
3. Request a new token using your original username and password, along with the tenant Id you want to access
Once you receive the impersonation token, any calls to the API will return data associated with requested TenantID, instead of the one related to your user.
Refreshing a token
When your access token expires, you will need to request a replacement token to continue accessing the API.
To avoid having to re-authenticate with username and password, instead you can re-authenticate using the refresh token provided to you.
REFRESH REQUEST
POST https://api.buildxact.com/oauth/token
Content-Type: application/x-www-form-urlencoded
Body: refresh_token=<
refreshtoken>
&grant_type=refresh_token&client_id=
<clientid>
&client_secret=
<clientsecret>
Body Parameters
<refreshtoken> Refresh token value received when obtaining access token
<clientid> API client id as provided by Buildxact
<clientsecret> API client secret as provided by Buildxact
This will provide you with a new access token, and a new refresh token as well. By storing the new refresh token as a replacement of the old one, you can ensure that your application stays logged in and never needs to use the username/password again.
2. Third-Party (Delegated) Authentication
With third-party authentications, the user is taken to a consent screen where they can see which scopes the Application (Third-Party application) is interested in accessing (Such as Catalogues, Leads etc.) and they have the choice to Accept or Deny the request. Once they grant consent, an auth code is returned to the consumer, which they can then use to get a token and a refresh token for accessing our platform. The token is specific to the user granting access. Only information for that user would be available, and additionally, the scopes would limit which APIs the consumer can call on behalf of the user. This flow is suitable for consumers (third-party application) that don’t have direct access to the Buildxact application, but their users do and the consumers want to work with data on behalf of the user. This flow would allow you to build a per user integration for each user that has consented to granting access to your application (referred to as Third-Party\Delegated application).
You can learn more about the user consent and third-party applications here.
Register as a Third-Party Application
You first need to contact Buildxact support and request to be registered as a Third-Party Application.
You will need to provide Company\Application Name(Required, shown on the consent screen), Logo (Optional, shown on the consent screen) and Redirect Urls.
To understand the redirect urls, you need to read the sections below and based on how your company sets up the third-party application flow, you can then send the redirect urls to Buildxact support alongside the other details.
You will then receive a Client Id and Client Secret which you'll need to use in the auth flow (described below).
Delegated Flow Setup
This flow consists of multiple steps that you have to execute in order for the auth code to be returned to your application, which you can then use to retrieve a Token\RefreshToken for the specified user.
Step 1: Point the user to our oauth2 page which will check if the user is logged in or not. If the user is logged in, they have to select some information within our app and then the call is redirected back to your application. If the user is not logged in, they first must log in and then the flow of the logged in process takes places.
You can do this by forwarding the user to the following page (url):
https://app.buildxact.com/oauth2.html?redirectUrl={{REDIRECT_URL}}
The RedirectUrl is the page where you will receive a bxcontext, and where you will setup the Third-Party\Delegated Consent flow. The redirect url should not contain any query string parameters - it should be a plain url such as: app.sample.com/redirectUrl
. This url must match one of the redirect urls you have asked Buildxact support to configure against your integration.
Once our app executes the process described above, the user will be redirected back to the RedirectUrl and it will contain a bxcontext query string parameter. You will later need to provide this when initializing the consent screen.
Step 2: This is the page that you will use in the RedirectUrl and has to be setup in the Third-Party app RedirectUrls (contact support).
On this page you will open the consent screen through auth0 - you can redirect the user to the full url constructed below, and you must provide a redirect_uri (replace the placeholder with the actual values you have):
Parameters description:
Step 3: Once you get the code on the RedirectUri auth0 responds to, which would be in a query string parameter called code, use that code to call auth0 to get a Token\RefreshToken for the user. You can only use the code once. You can learn more about the code flow here.
Make a Post request to the following url:
https://login.buildxact.com/oauth/token
with the following request body:
Parameter Details:
A successful response would contain an access_token, refrest_token, expires_in (seconds), as shown below:
It is recommended that you store the access_token and the refresh_token (for each combination of user and bxcontext separately, as they are based on the user), as you'd need them for future use, as described in the section below.
Refresh Token
You can utilize the refresh token to keep regenerating the token for the user. You can learn more about the refresh tokens here. You must also include the bxcontext when refreshing the token.
Post to /oauth/token
, same as in Step 3 where you post with the authorization code, but now with the following body:
All of these parameters are described above. BxContext is the same value mentioned in Step 2.
Response:
Store\Use the access_token. You can use the same refresh_token to regenerate a new access_token as many times as you'd like (as long as the refresh_token is active, more info on refresh tokens can be found here). A new refresh token is generated only when the use grants access again (if they follow the same flow described above from start to finish).
Revoke Access
The users can Revoke access at any time through the Buildxact application. This will cause the calls to regenerate the token and to the Api to fail.